Configuring the Loopback Device Limit The maximum number of loopback devices in Red Hat Enterprise Linux 6 is set by the maxloop kernel option. For example, to set the maximum number of loopback devices to 64, edit the /etc/grub.conf file, and add maxloop=64 at the end of the kernel line. 2.1.2 Mon Aug 11 2014 2.1.1 Fri Aug 08 2014 2.1.0. LoopBack is a mobile backend framework that you can run in the cloud or on-premises.
This tutorial walks you through the MySQL connector implementation to teach youhow to develop a connector for relational databases.
Understand a connector's responsibilities
In LoopBack, models encapsulate business data and logic as JavaScript propertiesand methods. One of the powerful features of LoopBack is that application developers don't have to implement all behaviors for their models as a lot of them are already provided by the framework out of box with data sources and connectors. For example, a model automatically receives the create, retrieve, update, and delete (CRUD) functions if it is attached to a data source for adatabase. LoopBack abstracts the persistence layer and other backend services, such as REST APIs, SOAP web services, and storage services, and so on, as data sources, which are configurations of backend connectivity and integration. Each data source is backed a connector which implements the interactions betweenNode.js and its underlying backend system. Connectors are responsible for mapping model method invocations to backend functions, such as database operations or call to REST or SOAP APIs. The following diagram illustrates howconnectors fit into the big picture of LoopBack API framework.
Please note that you don't always have to develop a connector to allow your application to interact with other systems. Ad-hoc integration can be done with custom methods on the model. The custom methods can be implemented using other Node modules, such as drivers or clients to your backend.
You should consider to develop a connector for common and reusable backend integrations, for example:
- Integrate with a backend such as databases
- Reusable logic to interact with another system
There are a few typical types of connectors based on what backends they connectto and interact with.
- Databases that support full CRUD operations
- Oracle, SQL Server, MySQL, Postgresql, MongoDB, In-memory DB
- Other forms of existing APIs
- REST APIs exposed by your backend
- SOAP/HTTP web services
- Services
- Push notification
- Storage
The connectors are mostly transparent to models. Their functions are mixed intomodel classes through data source attachments.
Most of the connectors need to implement the following logic:
Lifecycle handlers
- initialize: receive configuration from the data source settings and initialize the connector instance
- connect: create connections to the backend system
- disconnect: close connections to the backend system
- ping (optional): check if the connectivity
Model method delegations
- Delegating model method invocations to backend calls, for example CRUD
Connector metadata (optional)
- Model definition for the configuration, such as host/url/user/password
- What data access interfaces are implemented by the connector (the capability of the connector)
- Connector-specific model/property mappings
To mixin methods onto model classes, a connector must choose what functions tooffer. Different types of connectors implement different interfaces that groupa set of common methods, for example:
Database connectors
- CRUD methods, such as create, find, findById, deleteAll, updateAll, count
E-mail connector
- send()
Storage connector
- Container/File operations, such as createContainer, getContainers, getFiles,upload, download, deleteFile, deleteContainer
Push Notification connector
- notify()
REST connector
- Map operations from existing REST APIs
SOAP connector
- Map WSDL operations
In this tutorial, we'll focus on building a connector for databases that providethe full CRUD capabilities.
Understand a database connector with CRUD operations
LoopBack unifies all CRUD based database connectors so that a model can chooseto attach to any of the supported database. There are a few classes involved here:
PersistedModelClass defines all the methods mixed into a model for persistence.
The DAO facade maps the PersistedModel methods to connector implementations.
CRUD methods need to be implemented by connectors
In the next sections, we will use MySQL connector as an example to walk throughhow to implement a SQL based connector.
Define a module and export the initialize function
A LoopBack connector is packaged as a Node.js module that can be installed usingnpm install
. LoopBack runtime loads the module via require
on behalf of data source configuration, for example, require('loopback-connector-mysql');
. The connector module should export an initialize
function as follows:
After the initialization, the dataSource object will have the following properties added:
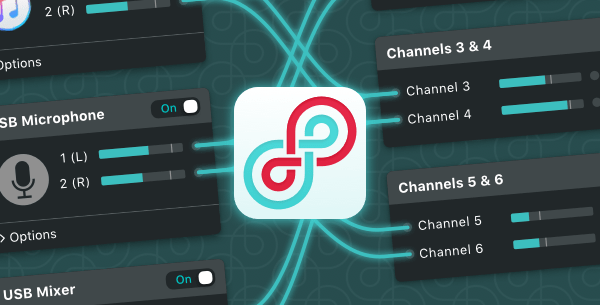
- connector: The connector instance
- driver: The module for the underlying database driver (
mysql
for MySQL)
The initialize
function should calls the callback
function once the connectorhas been initialized.
Create a subclass of SqlConnector
Connectors for relational databases have a lot of things in common. They are responsible for mapping CRUD operations to SQL statements. LoopBack provides abase class called SqlConnector
that encapsulates the common logic for inheritance.The following code snippet is used to create a subclass of SqlConnector.
Implement methods to interact with the database
A connector implements the following methods to communicate with the underlyingdatabase.
Connect to the database
The connect
method establishes connections to the database. In most cases, aconnection pool will be created based on the data source settings, includinghost
, port
, database
, and other configuration properties.
Disconnect from the database
The disconnect
method close connections to the database. Most database driversprovide APIs.
Ping the database
The ping
method tests if the connection to the database is healthy. Mostconnectors choose to implement it by executing a simple SQL statement.
Implement CRUD methods
The connector is responsible for implementing the following CRUD methods. The good news is that the base SqlConnector now have most of the methods implementedwith the extension point to override certain behaviors that are specific to theunderlying database.
To extend from SqlConnector, the minimum set of methods below must be implemented:
Execute a SQL statement with parameters
The executeSQL
method is the core function that a connector has to implement. Most of other CRUD methods are delegated to the query
function. It executes a SQL statement with an array of parameters. SELECT
statements will produce an array of records representing matching rows from the database while other statements such as INSERT
, DELETE
, or UPDATE
will report the number of rows changed during the operation.
Map values between a model property and a database column
Helpers to generate SQL statements and parse responses from DB drivers
Override other methods
There are a list of methods that serve as default implementations in the SqlConnector.The connector can choose to override such methods to customize the behaviors. Pleasesee a complete list at http://apidocs.strongloop.com/loopback-connector/.
Implement database/model synchronization methods
Loopback 4.0
It's often desirable to apply model definitions to the underlying relationaldatabase to provision or update schema objects so that they stay synchronizedwith the model definitions.
automigrate and autoupdate
Loopback 2.2.3
There are two flavors:
- automigrate - Drop existing schema objects if exist and create them based on model definitions. Existing data will be lost.
- autoupdate - Detects the difference between schema objects and model definitions, alters the database schema objects. Existing data will be kept.
The automigrate
and autoupdate
operations are usually mapped to a sequence of DDL statements.
Build a CREATE TABLE statement
Check if models have corresponding tables
Alter a table
Build column definition clause for a given model
Build index definition clause for a given model property
Build indexes for a given model
Build column definition for a given model property
Loopback 2 License Key
Build column type for a given model property
Implement model discovery from database schemas
Loopback 2
For relational databases that have schema definitions, the connector can implement the discovery capability to reverse engineer database schemas intomodel definitions.